本文共 795 字,大约阅读时间需要 2 分钟。
概述
在新车型的开发过程中需要对不同环境、不同工况下车辆进行功能性能的测试,确保车辆的各项指标能够满足开发要求。由于车辆传统的零部件测试以及“三高”测试无法兼顾系统集成、测试周期和成本,恒润科技携手国家汽车质量监督检验中心(北京通州)排放试验室提出一套整车高低温转毂电性能测试的解决方案,可在不同环境下对轻型汽车整车功能、性能进行验证评价,缩短整车开发周期,降低测试成本,提升车辆产品力。
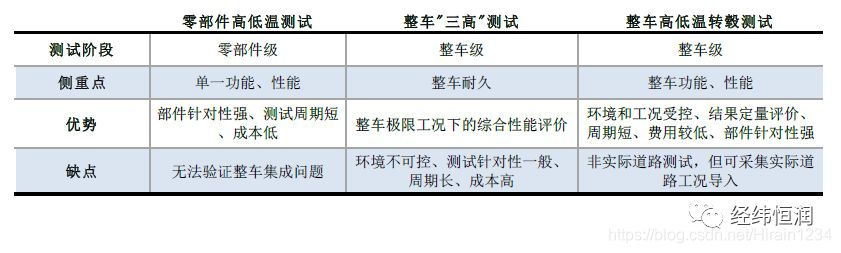
• 测试环境
国家汽车质量监督检验中心(北京通州)排放试验室具备完善的车辆测试环境和测试管理能力,试验室配置美国BURKE四驱底盘测功机、德国SCHENCK两驱底盘测功机、轻型汽车排放试验环境仓系统、轻型汽车冷起动及除霜除雾试验环境系统等多套专业测试设备,满足传统车辆、混合动力、纯电动车辆等多种车辆的测试需求。
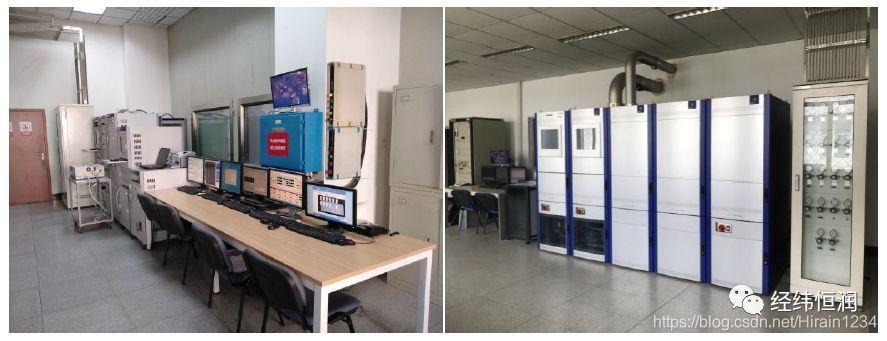
试验室控制管理系统
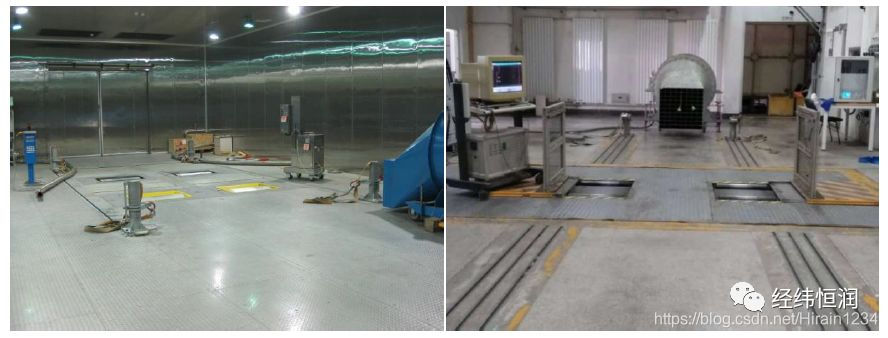
美国 BURKE四驱底盘测功机(左)、德国SCHENCK底盘测功机(右)
• 高低温转毂电性能测试服务
♦ 恒润电性能测试介绍
恒润科技电性能团队前后为30多家主机厂提供专业的工程咨询服务,实车测试工程经验丰富。测试涵盖12V/24V低压控制系统以及高压动力系统,能够针对整车低压电源系统、线束系统、接地系统、高压安规、高压性能等提供专业的解决方案和系统评估,提升车辆品质。
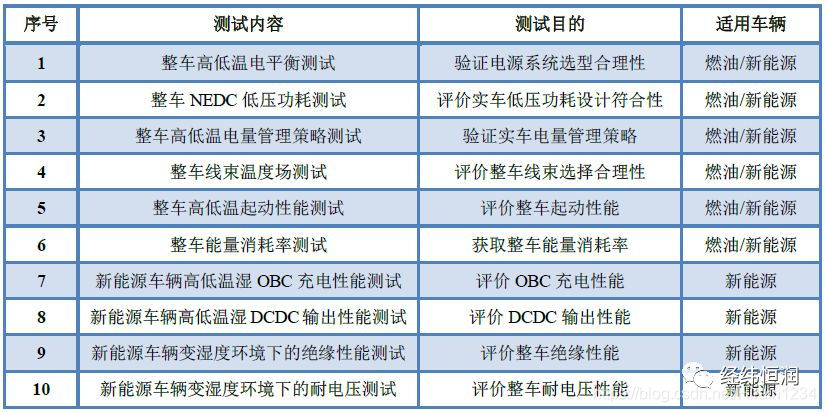
高低温转毂电性能测试内容
♦ 高低温转毂电性能测试
通过与国家汽车质量监督检验中心(北京通州)排放试验室深度合作,使测试车辆不仅能够快速达到并稳定在设定的温湿度,还能够按照特定行驶工况模拟路试。电性能测试可同步记录电压、电流、温度、总线等信号,测试软件支持数据回放、离线分析等功能,便于对测试车辆进行分析,形成测试报告,为后续车辆的开发提供数据支撑。因此,高低温转毂下的电性能测试可以完全满足主机厂对不同环境、不同工况下整车级“定量”测试分析的需求,有效提升整车开发效率。
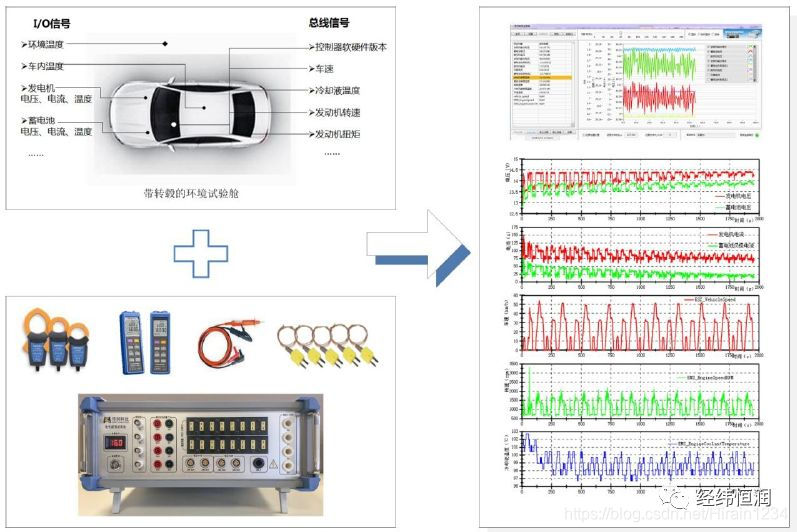
测试采集信息展示
转载地址:http://dbuj.baihongyu.com/